How to use REST API architecture to programmatically retrieve data from Flowfinity applications (Read)
Flowfinity allows programmatic access to views in applications. When access is enabled, programmers can use the HTTPS request/response-based REST API model to query view data in Flowfinity Actions.
This article outlines how to compose requests and which responses to expect based on a Lead Registration example app, which is designed to capture and manage sales leads. You can download an FTF for the Lead Registration app.
To enable REST access to app Operations, click the 'Configure' tab in the App Editor, and follow these steps.
Once access is enabled, the REST URL endpoint address for querying the view will be displayed in the Configure tab > Integration tab > REST Integration section. Select 'Details' to view the URL endpoint and an example of the JSON payload.
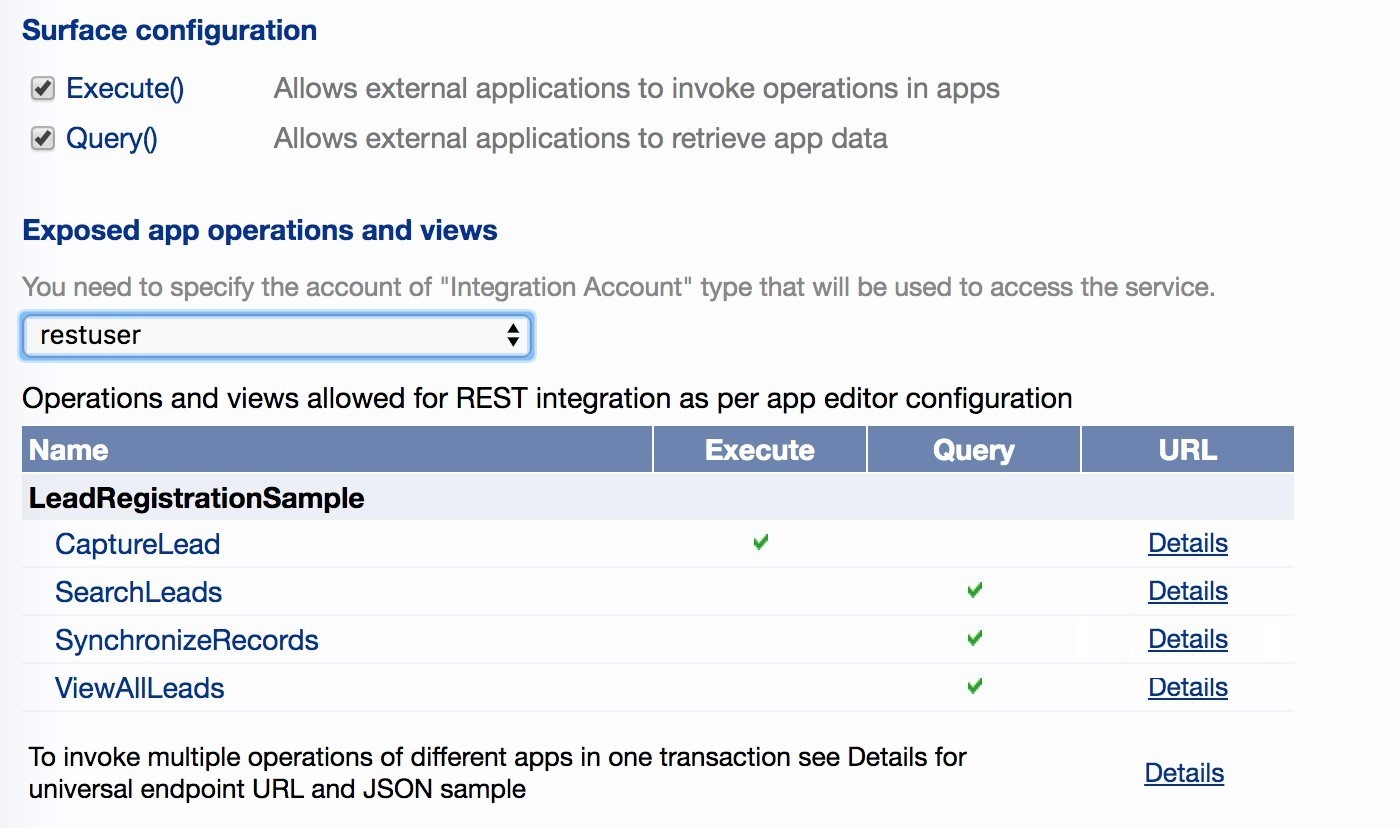
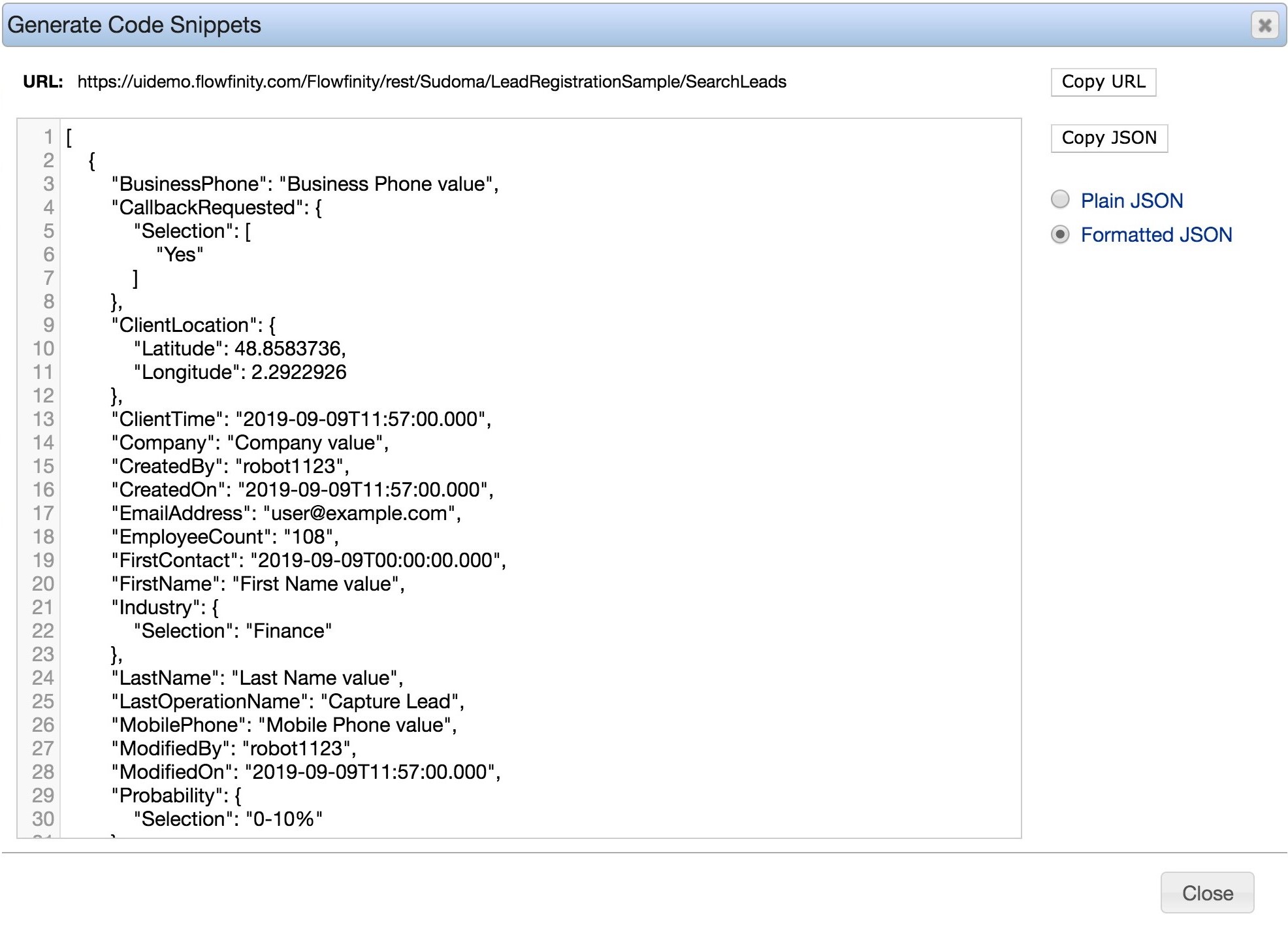
If the view has parameters, the parameter values should be encoded into the URL as name-value pairs.
Here is an example JSON operation format and explanations of the fields that Flowfinity accepts including specifics of how each of the app field types are transmitted, and considerations when using special system fields.
Instructions
View JSON format
Flowfinity Actions returns records of the view in a JSON array format with each record representing a separate JSON object. This is the JSON format for a Lead Registration Sample Search Leads query response:
[
{
"BusinessPhone": "555-7777",
"CallbackRequested": {
"Selection": [
"Yes"
]
},
"Company": "ABC Inc",
"EmailAddress": "jsmith@example.com",
"EmployeeCount": "25",
"FirstContact": "2020-09-06T00:00:00.000",
"FirstName": "John",
"Industry": {
"Selection": "Energy"
},
"LastName": "Smith",
"MobilePhone": "555-8888",
"Probability": {
"Selection": "50-80%"
},
"Rating": {
"Selection": "Warm"
},
"Summary": "Interested in the product. Get in touch in 2 weeks.",
"TotalValue": "99" ,
"RecordID": "000227544",
"CreatedBy": "Mike Smith",
"CreatedOn": "2020-09-06T04:37:37.000",
"State": "Default State",
"ClientTime": "2020-09-06T04:37:37.000",
"LastOperationName": "Capture Lead",
"ModifiedBy": "Mike Smith",
"ModifiedOn": "2020-09-06T04:37:37.000",
"ExternalTransactionId": "Flowfinity-892369569",
"LastSubmissionId":"6278150750"
},
{
"RecordID": "000227546",
.....
}
]
Where there may be system fields (if the user enabled including Record objects in response to the viewrequest in the view configuration):
"RecordID" is a unique ID assigned to the record.
"ModifiedBy", "ModifiedOn" are the name of the user who performed last record modification and the date/time.
"CreatedBy", "CreatedOn" is the name of the user who created the record and the date/time.
"ClientTime" is the time when the record was submitted from the client.
"LastOperationName" is the name of the last operation performed on the record.
"State" is the state that the record is currently in.
"ClientLocation" (optional) is the GPS coordinates of the location where the record was submitted.
"LastSubmissionId" is the unique ID of the submission. This value changes every time there is a new submission.
"ExternalTransactionId" (optional) is the unique ID of the transaction assigned to the record by the external system that initiated the submission.
The rest of the field names at the top-level are custom fields that are specific to the app. The names of the fields are retrieved from the respective 'Field name' value of the app field as specified in the App Editor. Depending on the field type, the value of the field may be in a slightly different format.
In our example:
"Company", "FirstName" and "LastName" are plain input fields. Their values in JSON are specified as quoted-string text.
"EmailAddress" is an email field. Its value in JSON is also in a quoted-string text format. However, it also must represent a valid email address. If the quoted text does not represent a valid email format, the value will be rejected with an error.
"BusinessPhone" and "MobilePhone" are phone fields with a value in JSON as quoted-string text. If the text inside quotes does not represent a valid phone format, the value will be rejected with an error.
"CallbackRequested" is a checkboxes field, which in JSON as specified and a JSON object (enclosed in curly brackets) with a single property 'Selection' with a value of an array []. The desired selected value(s) should be listed as quoted strings within the JSON array definition (in our case the only selected value we want in the 'Yes' choice). The value for the selection must match one of the predefined values in the app, or the submission will be rejected with an error.
"FirstContact" is a datetime field which is quoted-string text that must conform to the ISO 8601 date format. (For more details see iso.org or digi.com.)
"Industry","Rating", "Probability" are dropdown or radio button single-select fields, with values in JSON specified as JSON objects (enclosed in curly brackets {}) with a single property "Selection" containing the quoted string value for the desired selection. The value for the selection must match one of the predefined values in the app, or the submission will be rejected with an error.
"TotalValue", "EmployeeCount" are numeric fields where the values in JSON are non-quoted numbers. The number values will not have any decimal places for numeric fields in an app that does not support "decimal" values to avoid receiving an error upon submission.
"Summary" is a memo field where the value in JSON is specified as a quoted string of text.
Using Views to synchronize records between Flowfinity Actions and external systems
Views can be used to synchronize records from Flowfinity apps to external systems. This is done in a similar way to using a getTransaction SOAP API.
Using parameters, you can configure the View to retrieve only new records that require synchronization.
The sample app, Lead Registration, demonstrates a method for how to configure a View and Parameter to efficiently retrieve updated records.
This method uses the system field 'lastSubmissionId' which contains the unique ID of a record submission in Flowfinity. The View SynchronizeRecords is configured to return only records that have lastSubmissionId greater than the value supplied in the parameter. The resulting list of records is then sorted by the 'lastRecordId' in ascending order. This is equivalent to the order in which records were last modified.
The View is also configured to return 'Record objects,' overriding any Layout settings. This way each record will also return all system variables for synchronization.
The C# code sample shows how to retrieve the lastSubmissionId from the last record and save it to file, and how to pass its value as a parameter to the view on the next run.
Transactions for REST API
By default, the HTTP based APIs are non-transactional. As a result, the request BODY may be truncated, and the response may not arrive at the client, even after it was successfully transmitted by the server.
Unless these situations are handled properly, the system that relies on HTTP API will behave unpredictably. Potentially important data could be missing, incomplete data could be recorded, or record duplication could occur.
Flowfinity contains several built-in features to deal with inherently unreliable HTTP nature.
Each REST API request is validated to contain a fully formed and valid JSON body which starts and ends with matching curly brackets {}. If it detects incorrect JSON the request will abort further execution and respond with an error.
The first thing to do when receiving the response from Flowfinity Actions is to parse the response to validate that it contains a valid JSON object. This will ensure that you are not losing any data.
Next, each record that you receive in response has two unique identifiers that can be used for filtering for duplicate records.
These identifiers are: 'RecordID' and 'LastSubmissionId.'
'LastSubmissionId' is a unique ID of the specific record operation submission. When a new operation is submitted for the record, its 'lastSubmissionId' will change.
'RecordID' is the unique identifier of the record in the system, it stays the same regardless of operation, or edits made to the same record.
To implement this strategy, save the 'LastSubmissionId' with the record. When a new update for the record is received, the 'lastSubmissionId' will be different.
You can download sample console application source code which shows how to submit the JSON and process results written in .NET C# language.