How to use REST API architecture to programmatically invoke operations in Flowfinity (Write)
Introduction
Flowfinity provides the ability to integrate with external systems using the HTTPS request/response based Representational State Transfer (REST) Application Programming Interface (API) by allowing programmatic access to invoke operations in Flowfinity applications.
This article describes how to compose requests and which responses to expect using a sample "Lead Registration" app. The sample app is designed to capture and manage sales leads and includes some common data capture fields. You can download an FTF of the app to import into your Flowfinity site.
Please enable REST access to app operations in the App Editor under the 'Configure' tab by following these steps.
Once access is enabled the REST URL endpoint address for executing operations will be displayed in the Configure > Integration > REST Integration section when selecting the 'Details' link along with an example of the JavaScript Object Notation (JSON) payload.
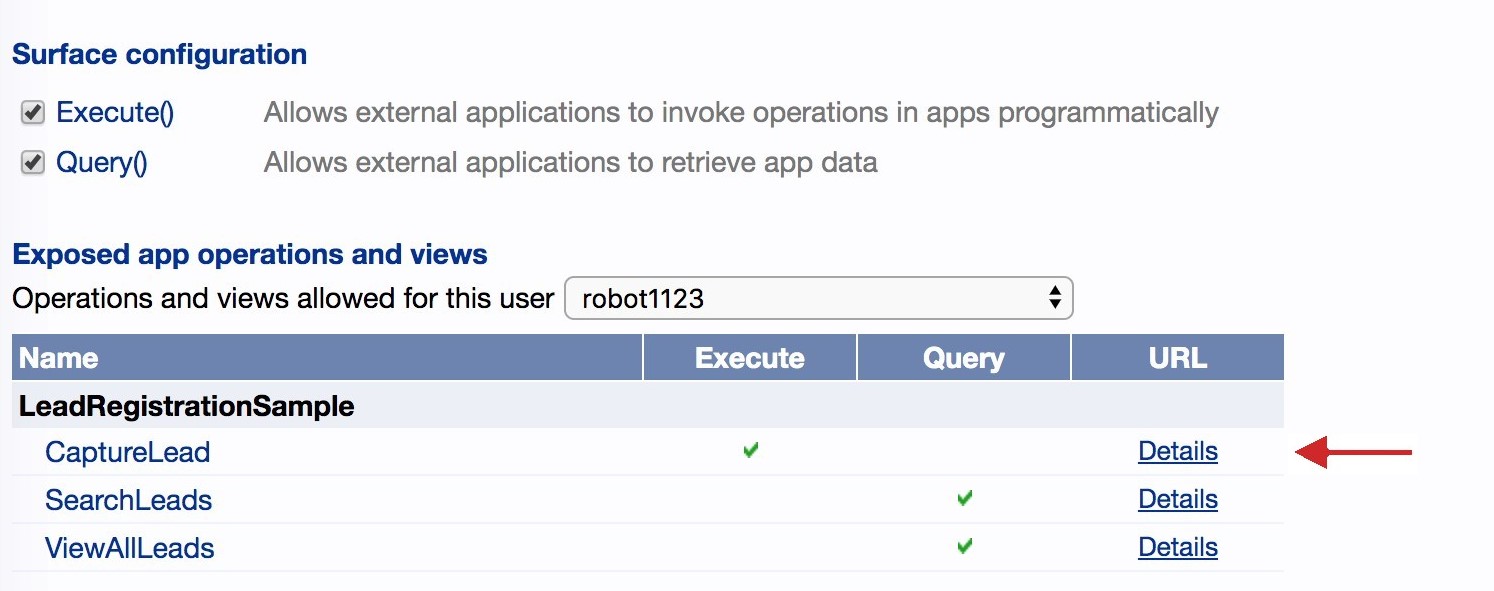
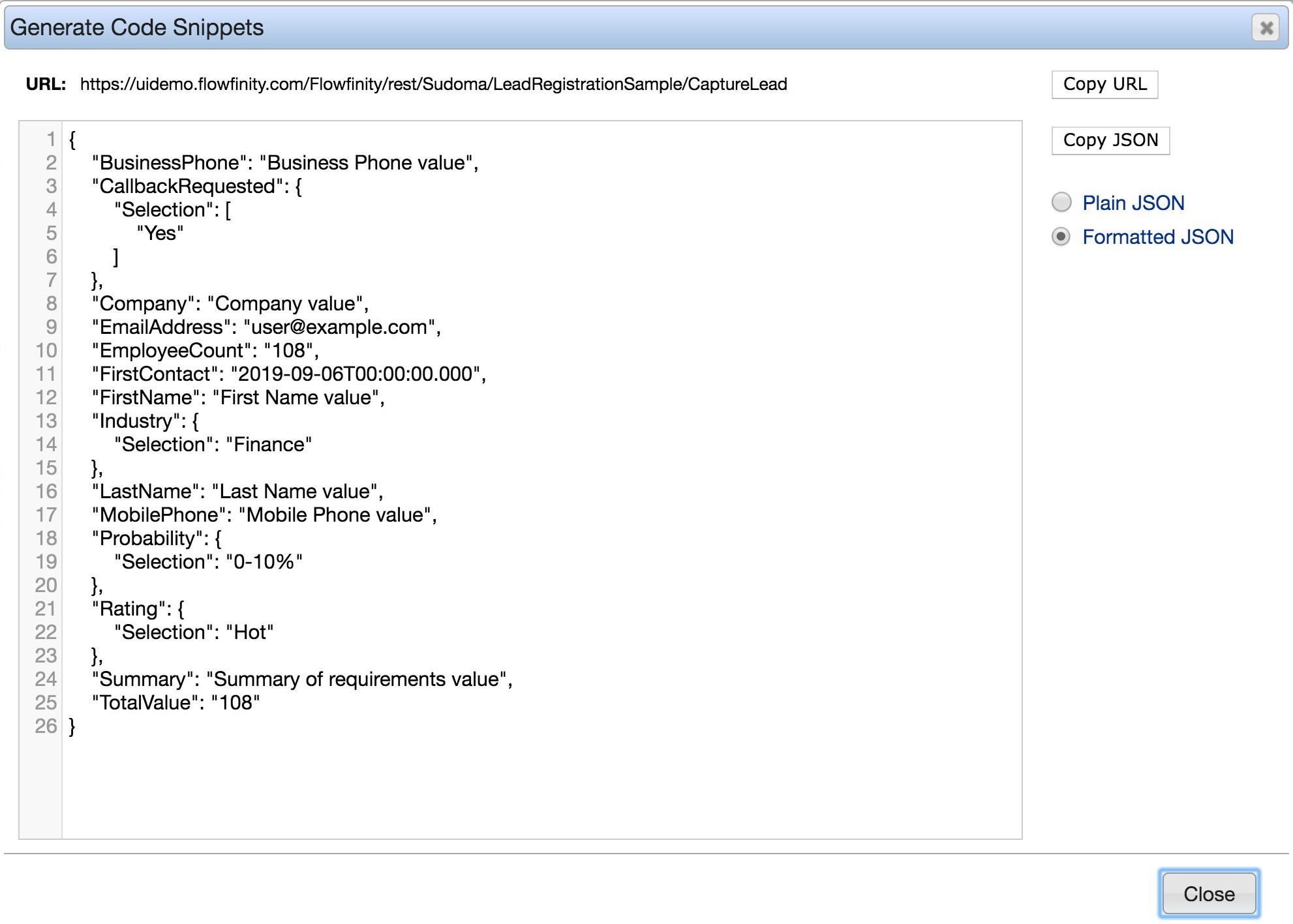
The benefit of using REST API architecture is that it is compatible with workflow orchestration cloud platforms such as Zapier and Power Automate (formerly Microsoft Flow). It can also be easily accessed from any development language by simply wrapping a JSON text string into an HTTPS POST request without the need to create custom classes or helpers as it is done with Simple Object Access Protocol (SOAP).
Here is an example JSON operation format and explanations of the fields that Flowfinity accepts including specifics of how each of the app field types are transmitted, and considerations when using special system fields.
Instructions
Operation JSON format
To invoke a Capture Lead operation in our app Flowfinity endpoint expect JSON like this:
{
"RecordID": null,
"ExternalTransactionId": null,
"Company": "ABC Inc",
"FirstName": "John",
"LastName": "Smith",
"EmailAddress": "jsmith@example.com",
"BusinessPhone": "555-7777",
"MobilePhone": "555-8888",
"CallbackRequested": {
"Selection": ["Yes"]
},
"FirstContact": "2020-09-06T15:44:04.6710234+03:00",
"Industry": {
"Selection": "Energy"
},
"Rating": {
"Selection": "Warm"
},
"TotalValue": 99,
"EmployeeCount": 25,
"Probability": {
"Selection": "50-80%"
},
"Summary": "Interested in the product. Get in touch in 2 weeks."
}
Where:
"RecordID" is an optional ID that will be assigned to the new record. If specified it must have a unique value. The value is optional for Add operations, as Flowfinity will assign a uniquely generated ID to the record automatically. This value is required for operations that operate on existing records, such as Edit, Delete, Transition, etc.
"ExternalTransactionId" is an optional ID that identifies the transaction. For more details on using this attribute see the 'Transactions in REST API' section of this article below.
The rest of the field names in the top level are custom fields that are specific to the app. The names of the fields are retrieved from the respective 'Field name' value of the app field as specified in the App Editor. Depending on the field type the value of the field may be in a slightly different format.
In our example:
"Company", "FirstName", "LastName" are plain input fields where values in JSON are specified as quoted string text.
"EmailAddress", is an email field, where value in JSON is specified as quoted string text which must also represent a valid email address. If the text inside the quotes does not represent a valid email format the value will be rejected with an error.
"BusinessPhone", "MobilePhone" are phone number fields where the value in JSON is also specified as quoted string text which must also represent a valid phone number. If the text inside the quotes does not represent a valid phone number format the value will be rejected with an error.
"CallbackRequested" is a checkbox field, which in JSON is specified as a JSON object (enclosed in curly brackets {}) with the single property “Selection” and value of an array []. The desired selected value(s) should be listed as quoted strings within the JSON array definition (in our case the only selected value we want is the “Yes” choice). The value for the selection must match one of the predefined values in the app, or the submission will be rejected with an error.
"FirstContact" is a datetime field which is a quoted string that must conform to the date format ISO 8601 (For more details see iso.org or digi.com)
"Industry", "Rating", "Probability" are dropdown or radio button single-select fields, with values in JSON specified as JSON objects (enclosed in curly brackets {}) with a single property “Selection” containing the quoted string value for the desired selection. The value for the selection must match one of the predefined values in the app, or the submission will be rejected with an error.
"TotalValue", "EmployeeCount" are numeric fields where the values in JSON are non-quoted numbers. The number values will not have any decimal places for numeric fields in an app that does not support “decimal” values to avoid receiving an error upon submission.
"Summary" is a memo field where the value in JSON is specified as a quoted string of text.
Omitted fields are treated as not included, resulting in default values being assigned to the field as per the default value configuration in the layout. To specify an empty string value, use the JSON string 0 length (“”). To reset a value to null for any type use a special string as a value “null” (in quotes) in the field.
Once the JSON is formed it needs to be sent as an HTTP(S) POST to the URL that can be obtained from the Details screen.
Operation JSON response
Flowfinity Actions will process the request, validate it, and based on the result will return back an HTTP(S) response with the respective HTTP code and JSON payload. You need to check the HTTP status code returned to filter for standard HTTP connectivity errors and critical server errors including any related to the data transmitted in the request (such as data validation failed).
If the status code is equal to or greater than 400 it means an error has occurred and the data was not applied. Data validation errors are returned by Flowfinity with status codes of 500. To find out more details about errors check the response HTTP header with name “x-error-message” which contains the text of the error returned. Once the error has been corrected you will need to re-submit the original request to save the data.
If the status code is 200 this means the request was successfully executed and the desired operation completed successfully. You can find request details such as the ExternalTransactionID, and system fields for the operation, including Record ID, in the Operations field. You may also optionally printout the updated record object including all final values (if enabled in the app Properties section of the App editor).
Here is an example JSON of the response:
{
"status": 200,
"ExternalTransactionId": "flowfinity-1567773941066"
“Operations”: [
{
"ClientLocation": {
"Latitude": 43.8583736,
"Longitude": 2.2922926
},
"ClientTime": "2020-09-09T11:26:00.000",
"CreatedBy": "robot1123",
"CreatedOn": "2020-09-09T11:26:00.000",
"LastOperationName": "Capture Lead",
"ModifiedBy": "robot1123",
"ModifiedOn": "2020-09-09T11:26:00.000",
"RecordID": "00000001",
"State": "Default State",
}
]
}
In the response, Operations array contains one object with list of all system variable values assigned to the records including RecordID.
Transactions for REST API
By default, the HTTP based APIs are non-transactional- which means that the request BODY may be truncated, and the response may not arrive to the client from the server after it was successfully processed. Unless these situations are handled properly the system that relies on the HTTP API will behave unpredictably, potentially missing data, recording incomplete data or duplicating records.
Flowfinity contains several built-in features to deal with inherent unreliability of HTTP:
Each REST API request is validated to contain a fully formed and valid JSON body which starts and ends with matching curly brackets {}. If it detects incorrect JSON the request will abort further execution and respond with an error.
Also, every REST request can have an optional 'ExternalTransactionId' value assigned which is highly recommended to be used with every operation call. The external transaction property can be any string text identifies the request. This property is used to detect duplicate operation calls which are typical when HTTP connectivity is interrupted between the server and the client after the server sent a successful response to the network, but there was a connectivity interruption and the response was not received by the client.
If the client resends the same operation quoting the same 'ExternalTransactionId' Flowfinty is designed to understand that this is a duplicate request, which it will ignore, and immediately return the response with HTTP code 200 and the JSON of the initial request that was executed.
To take advantage of this functionality the client code that sends the REST commands needs to implement special connection timeout error handling which upon receiving a connection timeout error (or any other desired failure errors) will resend the same transaction to Flowfinity.
You can find an example of this logic in the .NET sample referenced below where the code retransmits the operation with the same 'ExternalTransactionId' upon receiving error 404 or a connection timeout. In runtime this code will ensure that even if a connection timeout happened after the record was saved successfully in the database the data will not be duplicated.
Microsoft .NET C# sample source code:
You can download sample console application source code which shows how to submit the JSON and process results written in .NET C# language.